How to access Expressjs get params ?
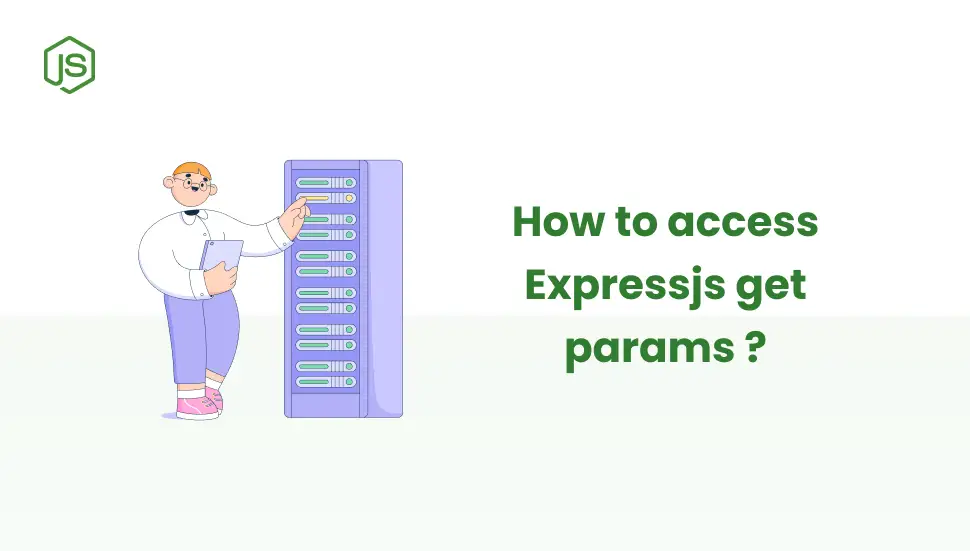
When building web applications with Express.js, you often need to work with data sent by users through URL parameters. These parameters, known as GET parameters, help you gather information from the URL itself.
This tutorial will guide you through accessing these parameters with clear and detailed steps.
Install Express.js
First, make sure you have Node.js and npm (Node Package Manager) installed on your machine. Then, create a new project directory and initialize it:
mkdir my-express-app
cd my-express-app
npm init -y
Now Let's Install Express.js by running:
npm install express
Set Up Your Basic Server
It's time to create a file named app.js
and set up a basic Express server for testing. copy and paste following code or type.
const express = require('express');
const app = express();
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
This code initializes an Express application and sets it to listen on port 3000
. Now, your server can handle incoming requests.
Create a Route with GET Parameters
To handle data sent through URL parameters, you need to create a route that listens for GET requests. Let’s create a route to capture user information, such as name
and age
, from the URL.
app.get('/user', (req, res) => {
const name = req.query.name;
const age = req.query.age;
if (!name || !age) {
return res.status(400).send('Both name and age parameters are required');
}
res.send(`Name: ${name}, Age: ${age}`);
});
In given code we,
First we have route /users
which is define in line app.get('/user', (req, res) => { ... })
to listens for GET requests on the /user
endpoint.
Then We Extract Parameters using req.query
which holds all GET parameters as key-value pairs.
We also have some validation to check ensures both parameters are provided; otherwise, it sends a 400 (Bad Request) response.
Finally we send a response back to the client using res.send
that contained the extracted parameters back to the client.
This setup ensures that your application responds properly whether the necessary parameters are present or not.
Test Your Route
In order to test our application first start your server by running:
node app.js
Open your browser and visit following address
http://localhost:3000/user?name=John&age=30
Now check your server terminal, you should see following output
Name: John, Age: 30
If you miss any parameter, and visit the following address :
http://localhost:3000/user?name=John
You will receive:
Both name and age parameters are required
Handling Multiple GET Parameters
You can also handle multiple parameters without any issues. For example, to capture additional parameters like email
and country
, we extend the route handler like below.
app.get('/user', (req, res) => {
const { name, age, email, country } = req.query;
if (!name || !age || !email || !country) {
return res.status(400).send('Name, age, email, and country parameters are required');
}
res.send(`Name: ${name}, Age: ${age}, Email: ${email}, Country: ${country}`);
});
rerun the server again and visit following url to test updated code.
http://localhost:3000/user?name=John&age=30&email=john@example.com&country=USA
You should see:
Name: John, Age: 30, Email: john@example.com, Country: USA
Tips for Organizing Your Routes
As your application grows, consider organizing your routes into separate files for easier maintenance.
For example, you can create a routes
directory and a user.js
file within it.
routes/user.js
const express = require('express');
const router = express.Router();
router.get('/', (req, res) => {
const { name, age, email, country } = req.query;
if (!name || !age || !email || !country) {
return res.status(400).send('Name, age, email, and country parameters are required');
}
res.send(`Name: ${name}, Age: ${age}, Email: ${email}, Country: ${country}`);
});
module.exports = router;
In your app.js
, incorporate the new route file:
const express = require('express');
const app = express();
const port = 3000;
const userRoutes = require('./routes/user');
app.use('/user', userRoutes);
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
This structure provides better organization and scalability for your application and it helps you to make your code more cleaner.
Conclusion
To access GET parameters in Express.js you can use req.query
build in feature in Express.js.