Using Axios to Post Form Data: A Comprehensive Guide
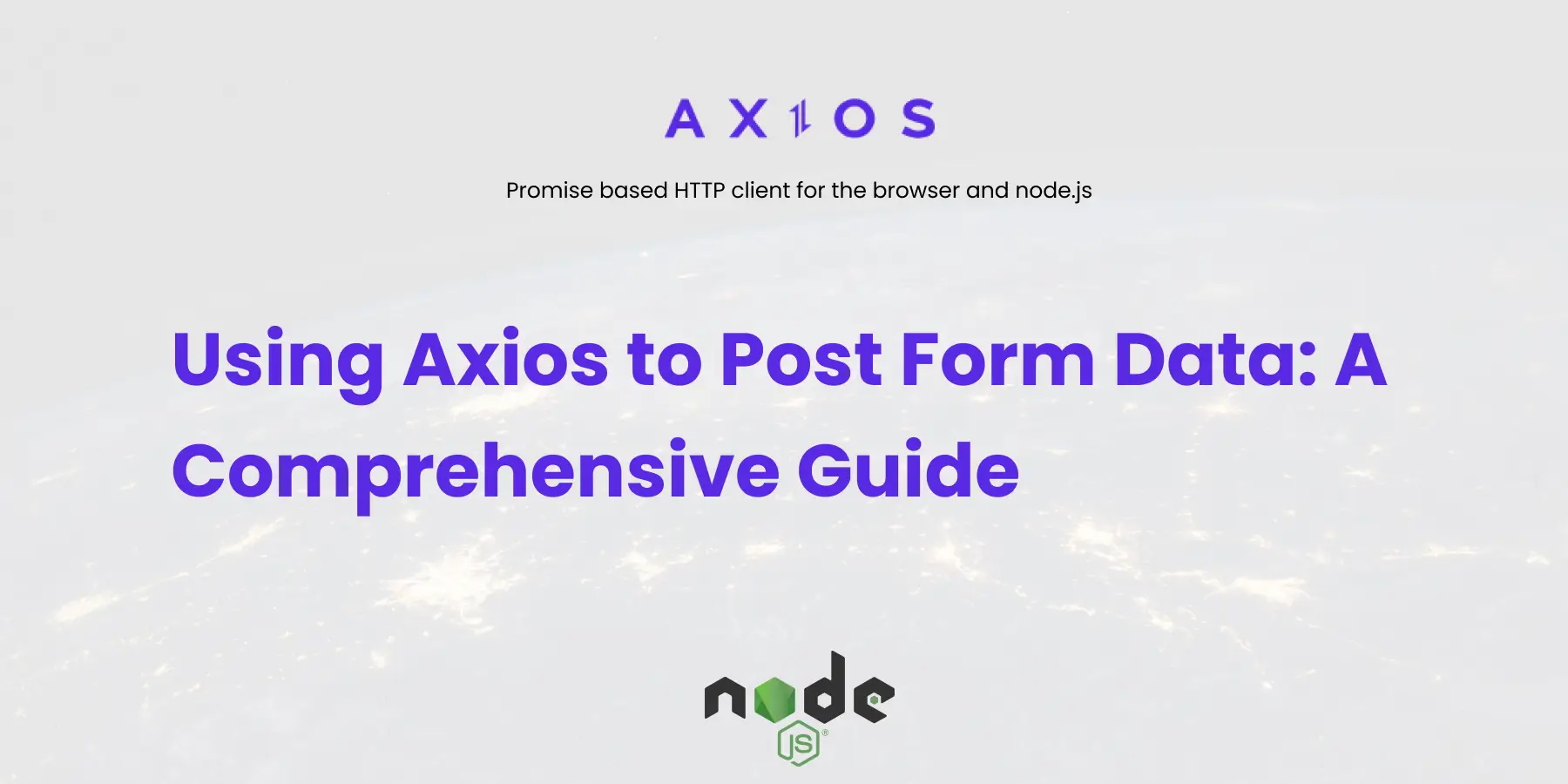
Axios is a popular JavaScript library used to make HTTP requests from web applications. Even though you can achieve the HTTP request sending using fetch
API which is build in JavaScript since Axios simplicity and ease of use developers are much like to use that.
Axios providing a clean and easy to use API for handling both GET and POST requests. It's also support older browsers as well. one common use case of Axios is sending form data to a server, and in this article, we'll learn how to effectively use Axios to post form data.
What is Axios?
First Let's have a look at what is Axios? and Why it's so useful?
Axios is a promise-based HTTP client for JavaScript, available for both the browser and Node.js which allows developers to make asynchronous HTTP requests to REST endpoints and perform CRUD operations.
Its key features include:
- Promise-based API: Makes it easy to work with asynchronous operations. (async await)
- Interceptors: Allows you to intercept requests or responses before they are handled by
.then()
or.catch()
. - Automatic JSON data transformation: Converts JSON data to JavaScript objects and vice versa.
- CSRF protection: Handles Cross-Site Request Forgery (CSRF) protection easily.
and Axios have following advantage over Fetch API
- Simple and Easy to understandable syntax - Axios is provide straightforward syntax when compare with fetch, which need to code lot of boiler plate code.
// GET request - Axios
axios.get('https://api.example.com/data')
.then(response => console.log(response.data))
.catch(error => console.error(error));
// GET request - fetch
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Fetch error:', error));
- Browser Capability - Axios supports older browsers, including Internet Explorer yet, Fetch is supported in most modern browsers but lacks support in Internet Explorer.
- Request and Response Interception - Axios has built-in support for request and response interception, allowing you to modify requests or handle responses globally:
- Transforming Request and Response Data - Axios automatically transforms JSON data to JavaScript objects and vice versa. But in fetch you have to manually transform data.
- Handling Timeouts - Axios allows you to easily set timeouts for requests, Fetch does not natively support timeouts.
- File Uploading - Axios makes handling file uploads simple with FormData
and there are more.
Now Let's have a look how to install Axios and use it for form data.
Installing Axios
Before using Axios, you need to install it. You can do this via npm or yarn:
npm install axios
or
yarn add axios
Posting Form Data with Axios
Posting form data with Axios is straightforward. Here’s a step-by-step guide to get you started:
Step 1: Create a Form
First, you need an HTML form. Here’s an example of a simple form:
<form id="user-form">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<button type="submit">Submit</button>
</form>
Step 2: Capture Form Data
Next, you need to capture the form data when the user submits the form. You can use JavaScript to listen for the form’s submit event and then collect the form data.
<script>
document.getElementById('user-form').addEventListener('submit', function(event) {
event.preventDefault();
const formData = new FormData(this);
// Logging form data to console for verification
for (let [key, value] of formData.entries()) {
console.log(`${key}: ${value}`);
}
// Call the function to post form data
postFormData(formData);
});
</script>
what we did here is addEventListner
to the our form submit and then get the for data as soon as after the form submission. then we call the postFormData
function which is responsible for sending form data.
Step 3: Post Form Data Using Axios
Now, you can use Axios to send the form data to your server.
<script>
function postFormData(formData) {
axios.post('https://your-endpoint.com/submit', formData)
.then(response => {
console.log('Form submitted successfully:', response.data);
})
.catch(error => {
console.error('Error submitting form:', error);
});
}
</script>
Here you can update the https://your-endpoint.com/submit
` url as you wish. and if you completed above steps now you can handle form data on the server.
Handling Form Data on the Server
Let's have a look how to handle sended data from Node.js with Express, But you able to handle those data from any back end.
Here we have used, expressjs and multer to handle form data. you can install those packages by following command.
npm init -y // skip if you already have project
npm install express multer
then create a index.js
file and paste following code
const express = require('express');
const app = express();
const multer = require('multer');
const upload = multer();
app.post('/submit', upload.none(), (req, res) => {
console.log(req.body);
res.send('Form data received!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Now run and your form data should be submitted to the backend and the data will be on console output.
Since your application running on port 3000, you can provide you back end url as http://localhost:3000/submmit
.
Extra Tips for Working with Axios
- Transform Data if Necessary: If you need to send the data in a specific format (e.g., JSON), you can transform the form data before sending it with Axios.
Example
const sendFormData = async (formElement) => {
const formData = new FormData(formElement);
// Transform the FormData object to a JSON object
const formObject = {};
formData.forEach((value, key) => {
formObject[key] = value;
});
- Error Handling: Always include error handling to manage any issues that may arise during the HTTP request.
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => {
// Handle successful response
console.log('Data:', response.data);
})
.catch(error => {
// Handle error
if (error.response) {
// The request was made and the server responded with a status code
console.error('Server responded with status:', error.response.status);
console.error('Response data:', error.response.data);
} else if (error.request) {
// The request was made but no response was received
console.error('No response received:', error.request);
} else {
// Something happened in setting up the request that triggered an error
console.error('Request failed:', error.message);
}
});
- CSRF Tokens: If your server uses CSRF tokens, make sure to include them in your Axios request headers.
const csrfToken = document.querySelector('meta[name="csrf-token"]').getAttribute('content');
axios.post('https://your-server-endpoint.com/submit', formData, {
headers: {
'X-CSRF-TOKEN': csrfToken
}
})
.then(response => {
console.log('Form submitted successfully:', response.data);
})
.catch(error => {
console.error('Error submitting form:', error);
});
Conclusion
If your application have form submissions Axios can be used to post form data is a simple and effective way. AS we mentioned in the article by using the Axios
you able to reduce your boilerplate code and by following the steps outlined in this guide, you can easily capture and send form data to your server, handle responses and handle errors gracefully.