20 Essential Built-In Nodejs Modules - You Can't Miss!
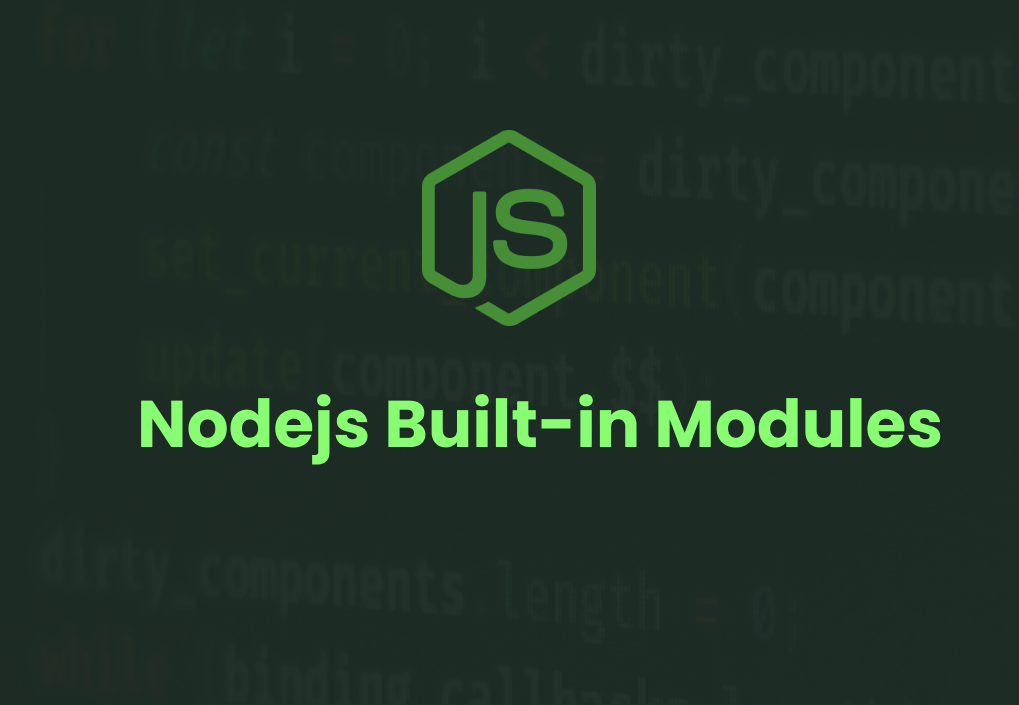
Most time we used to find packages from npm registry, But there are lot of useful built-In modules out of the box. Which help you to build your application easily.
Here are some 20 useful in-build modules in Nodejs.
List of Packages
- http
- https
- fs
- path
- os
- events
- stream
- util
- buffer
- querystring
- crypto
- url
- zlib
- dns
- child_process
- cluster
- timers
- net
- vm
- readline
http
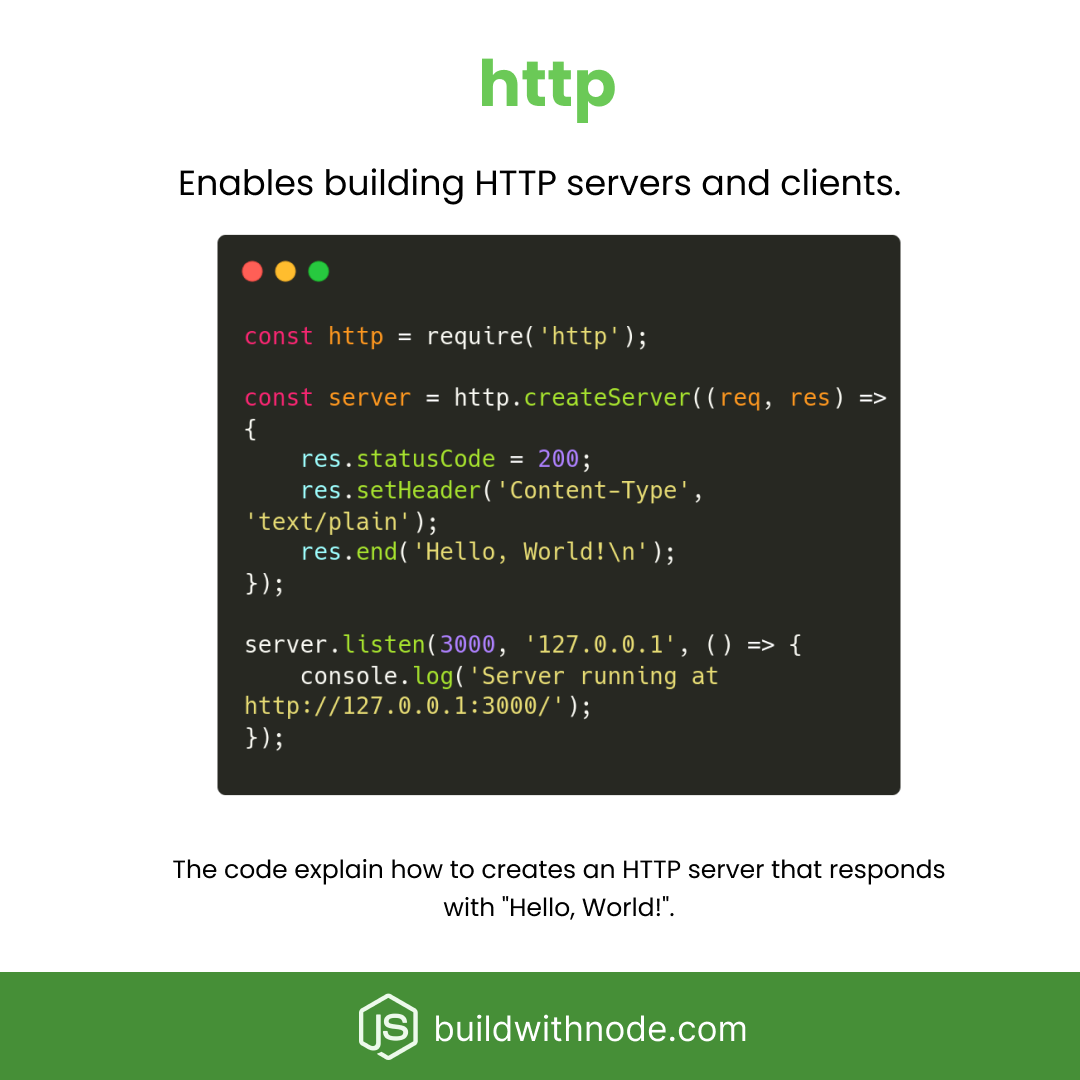
The http
module in Node.js allows you to create HTTP servers and clients. It provides methods for making HTTP requests and handling responses, making it possible to build web servers that can handle client requests, serve content, and manage data transfer over the web.
You can import http
module via require('node:http')
(CommonJS) or import * as http from 'node:http'
(ES module).
Here is a sample code how to build simple http server. follow the documentation to learn more about how to use http.
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
https
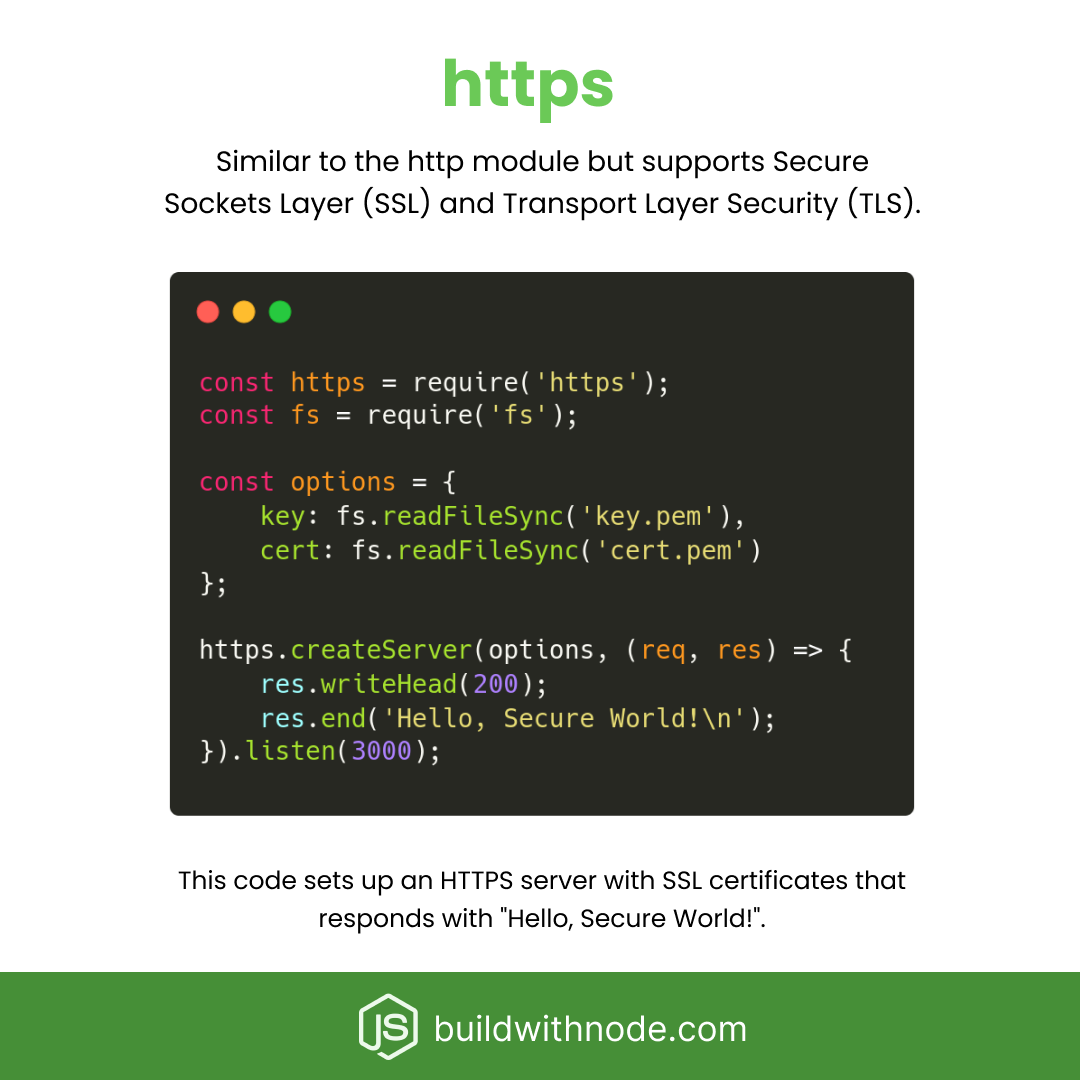
The https
module is similar to the http
module but includes support for Secure Sockets Layer (SSL) and Transport Layer Security (TLS).
This enables secure communication over the network by encrypting data, which is crucial for applications that require secure data transmission, such as e-commerce websites, banking applications, and other services that handle sensitive information.
If you are interested to learn more about https
module follow the documentation.
const https = require('https');
const fs = require('fs');
const options = {
key: fs.readFileSync('key.pem'),
cert: fs.readFileSync('cert.pem')
};
https.createServer(options, (req, res) => {
res.writeHead(200);
res.end('Hello, Secure World!\n');
}).listen(3000);
fs
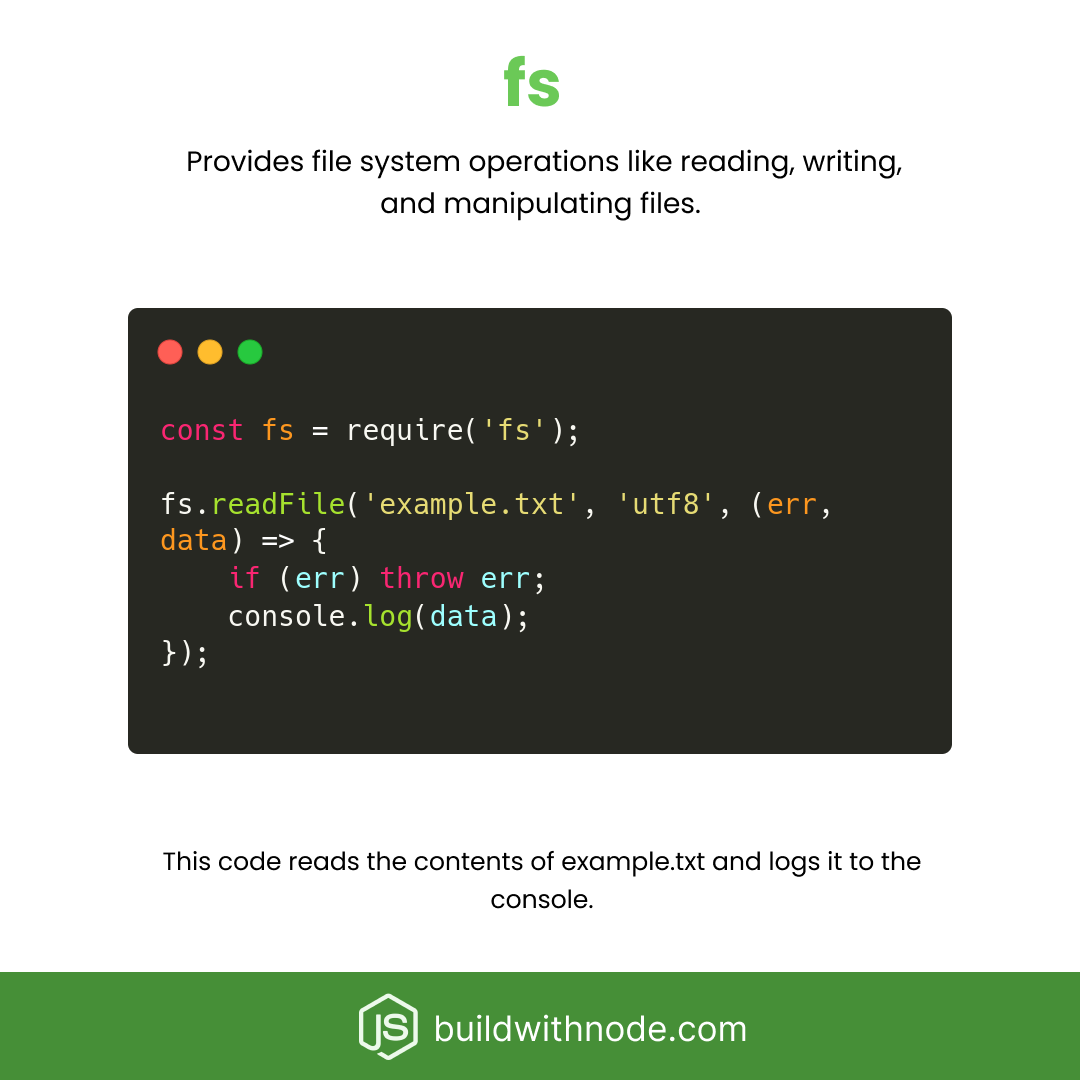
The fs (file system) module provides an API for interacting with the file system easily.
It enables operations like reading and writing files, creating and deleting directories, and modifying file permissions. This module is fundamental for server-side applications that need to handle file I/O operations, such as file uploads, downloads, and data storage.
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
path
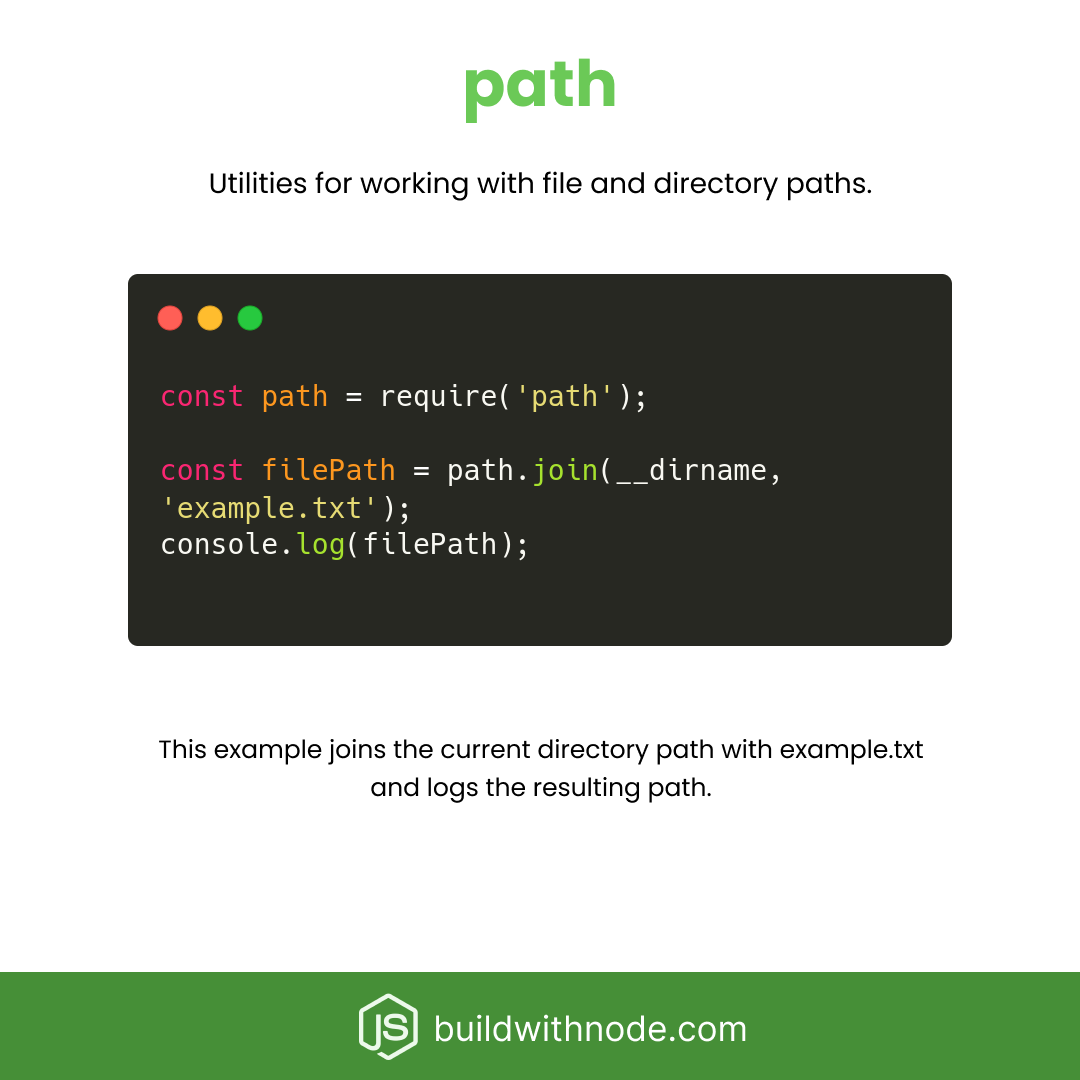
The path module provides utilities for working with file and directory paths.
It includes varies methods for manipulating paths, such as joining, resolving, and normalizing them, making it easier to handle file paths in a cross-platform manner. This module is particularly useful for file operations where path manipulation is necessary, ensuring that paths are correctly formatted regardless of the operating system.
Example code that who to use path module
const path = require('path');
const filePath = path.join(__dirname, 'example.txt');
console.log(filePath);
os
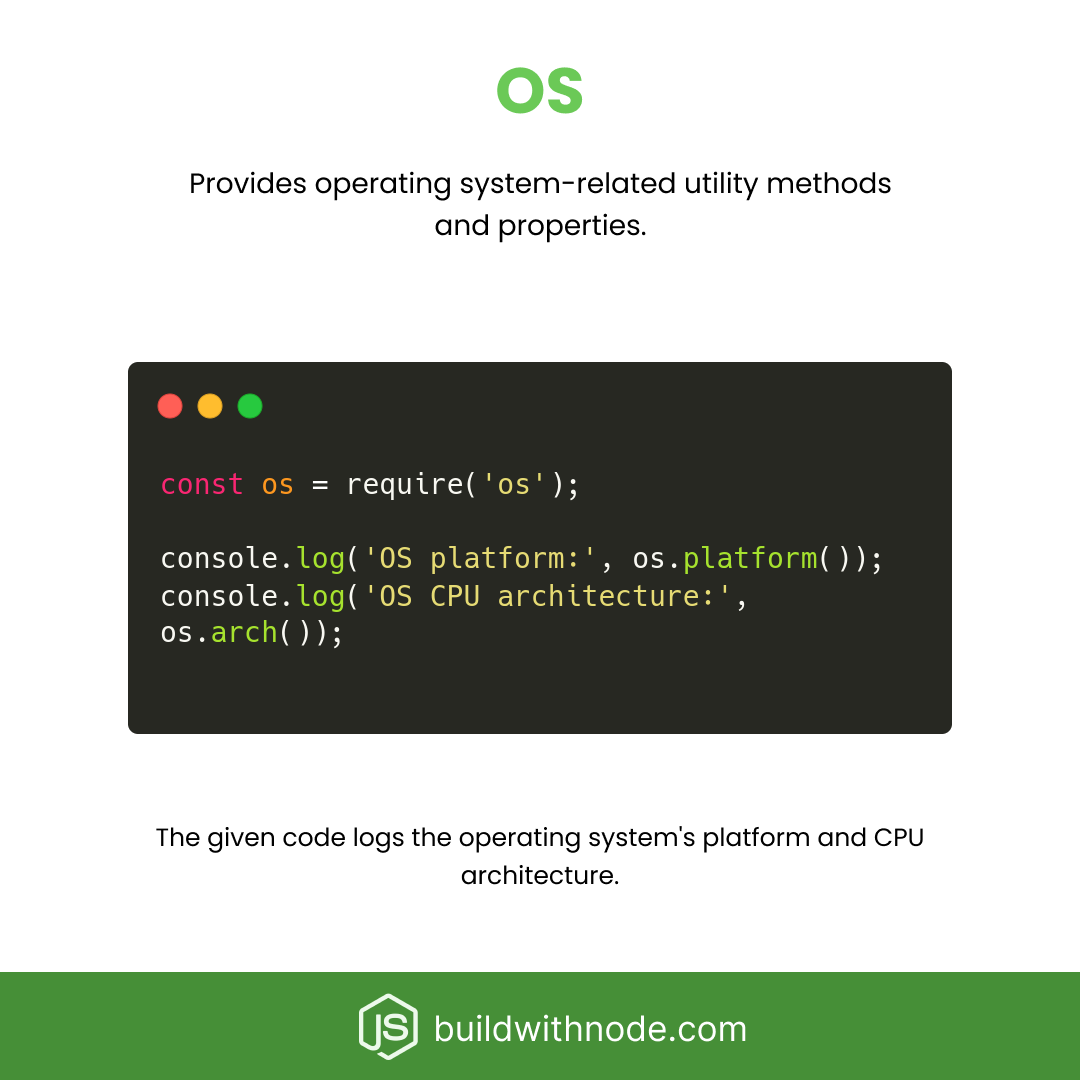
The os
module provides a number of operating system-related utility methods and properties.
It allows you to access information about the operating system, such as the hostname, platform, architecture, and available memory. This module is useful for applications that need to adapt based on the environment they are running in or need to provide system-level information and diagnostics.
const os = require('os');
console.log('OS platform:', os.platform());
console.log('OS CPU architecture:', os.arch());
events
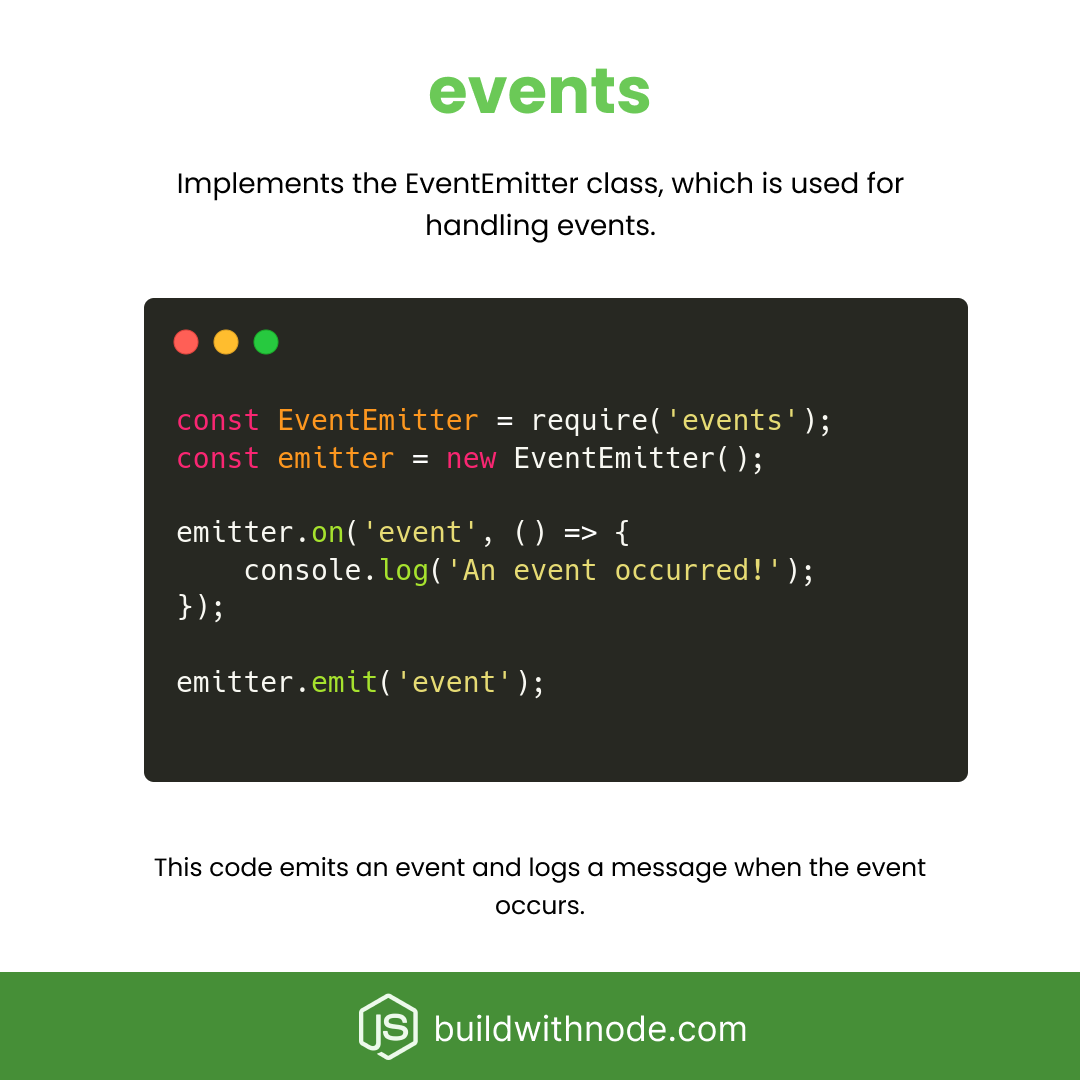
The events module implements the EventEmitter class, which is used for handling events in Node.js.
This module provides a mechanism for emitting and listening to events, enabling asynchronous programming and decoupling different parts of an application. It is widely used in Node.js for managing I/O operations, user interactions, and other asynchronous tasks.
const EventEmitter = require('events');
const emitter = new EventEmitter();
emitter.on('event', () => {
console.log('An event occurred!');
});
emitter.emit('event');
stream
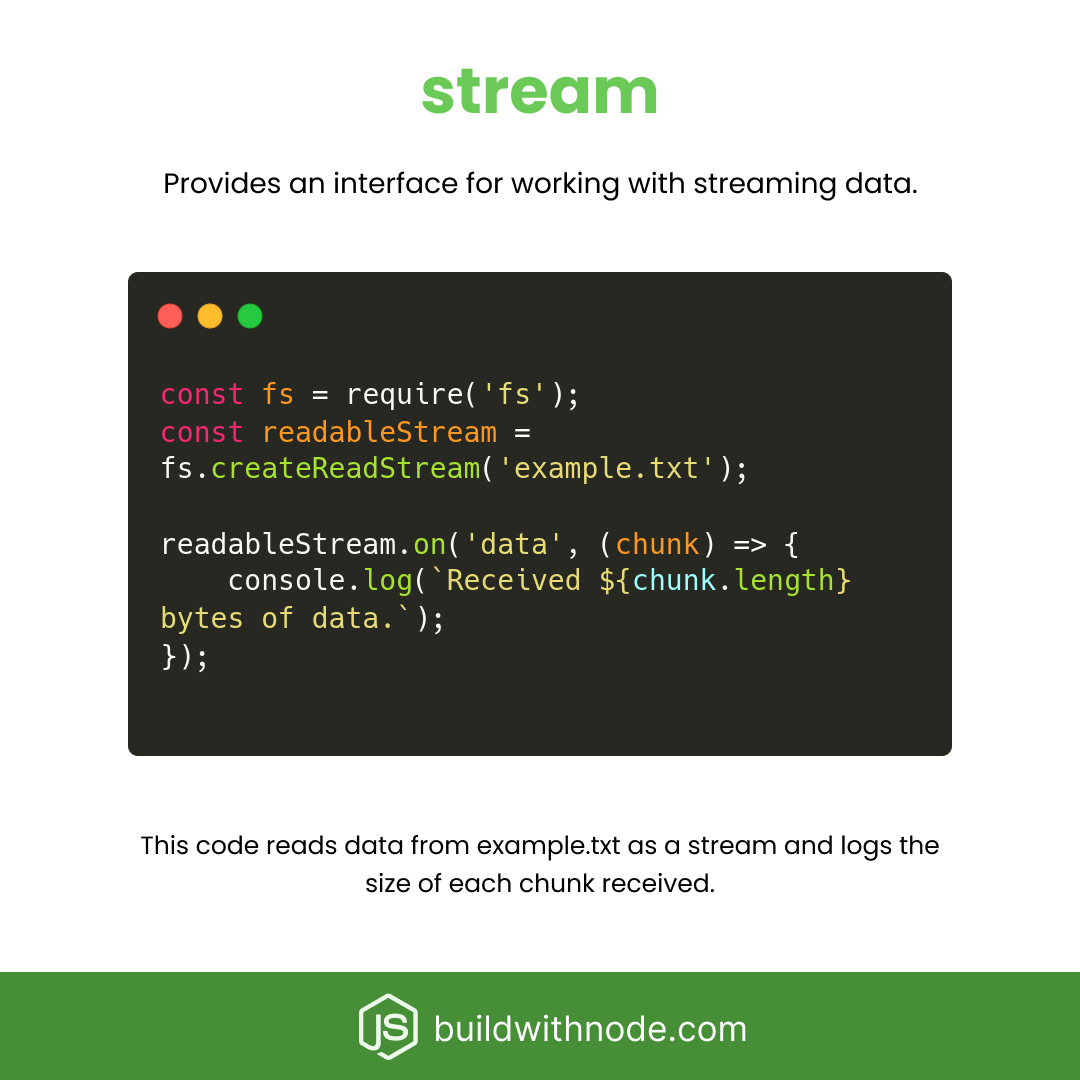
The stream module provides an API for working with streaming data easily.
Streams are used to handle reading and writing of data in a continuous manner, which is more efficient for handling large amounts of data or working with data that is being received or generated over time. This module is essential for tasks such as processing file uploads, streaming multimedia content, and handling network communications.
const fs = require('fs');
const readableStream = fs.createReadStream('example.txt');
readableStream.on('data', (chunk) => {
console.log(`Received ${chunk.length} bytes of data.`);
});
util
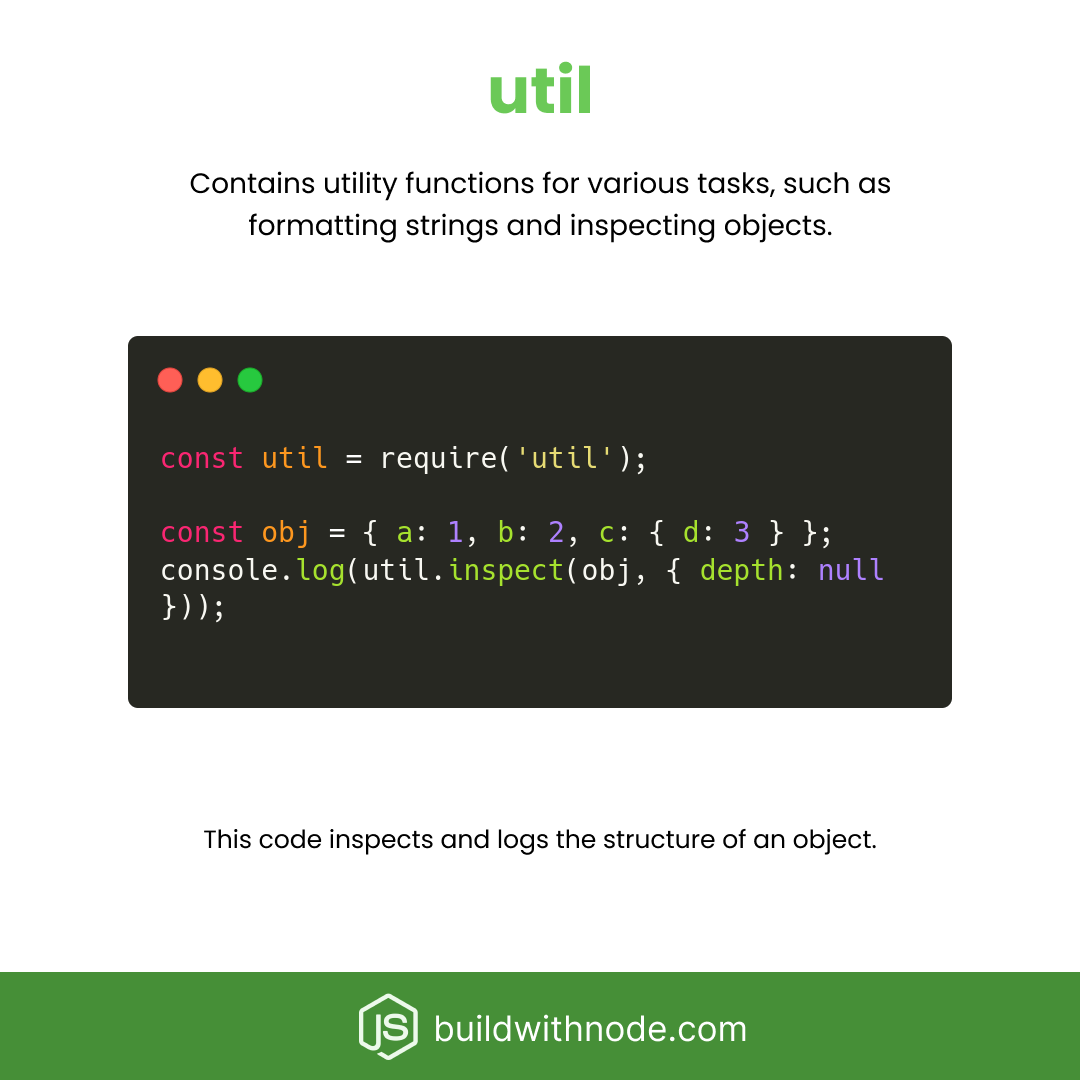
The util
module contains utility functions that help with various tasks in Node.js.
It includes methods for debugging, formatting strings, inheriting prototypes, and more. These utilities can simplify and streamline code by providing commonly needed functionalities, such as formatting console output and inspecting objects for debugging purposes.
const util = require('util');
const obj = { a: 1, b: 2, c: { d: 3 } };
console.log(util.inspect(obj, { depth: null }));
buffer
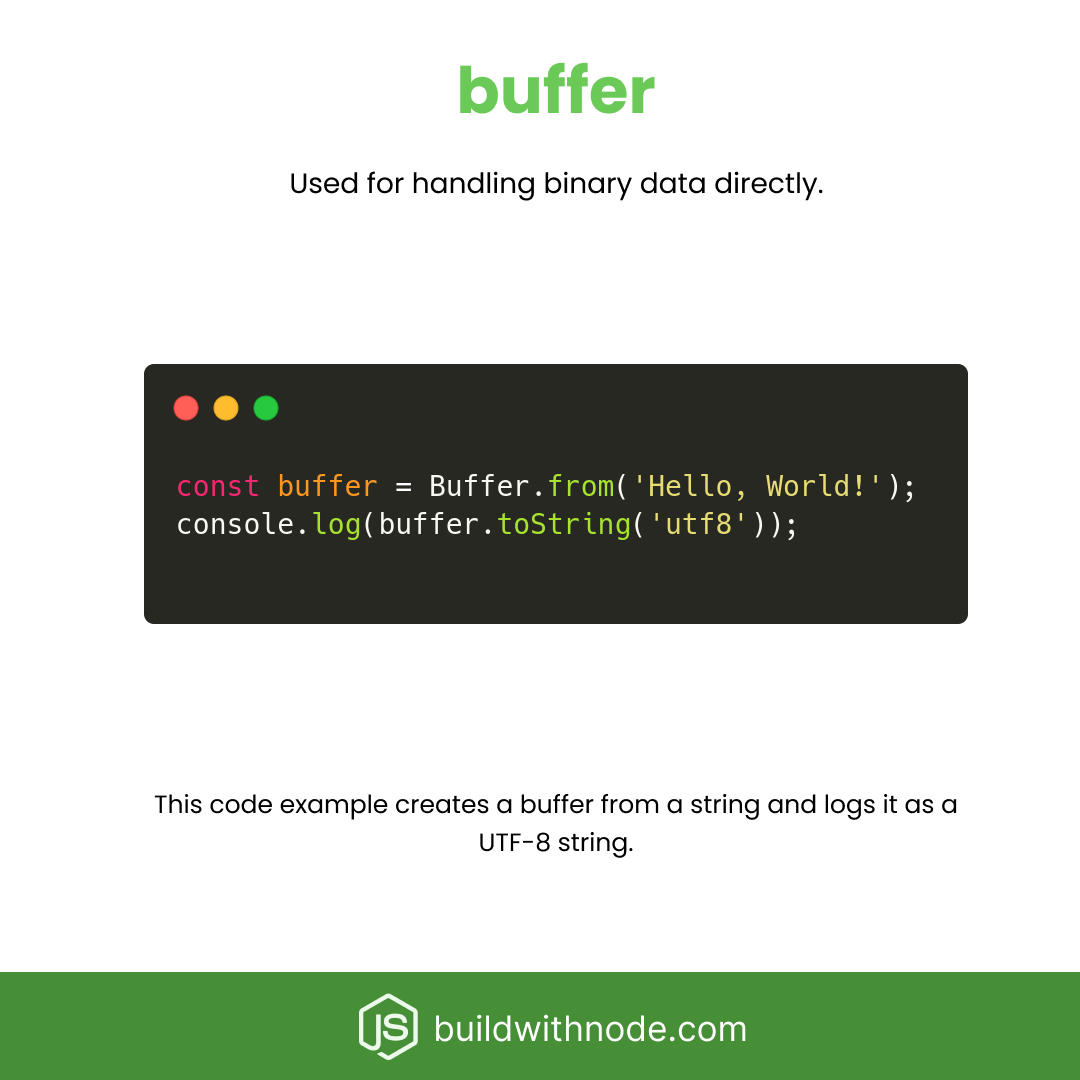
The util module contains utility functions that help with various tasks in Node.js. It includes methods for debugging, formatting strings, inheriting prototypes, and more. These utilities can simplify and streamline code by providing commonly needed functionalities, such as formatting console output and inspecting objects for debugging purposes.
const buffer = Buffer.from('Hello, World!');
console.log(buffer.toString('utf8'));
querystring
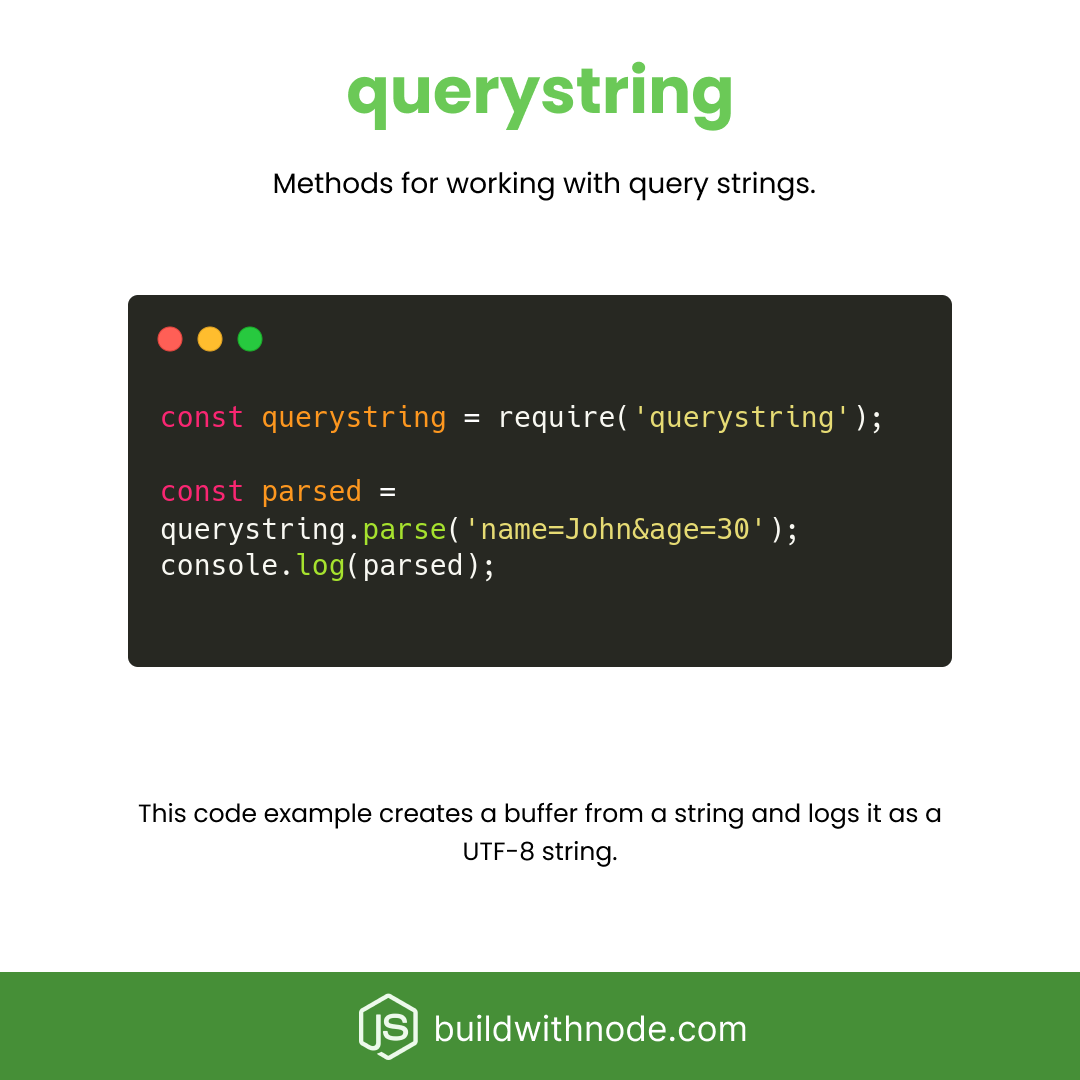
The querystring module provides utilities for parsing and formatting URL query strings.
It can convert query strings into objects and vice versa, making it easier to work with query parameters in web applications. This module is particularly useful for handling URL-encoded form data and constructing query strings for HTTP requests.
const querystring = require('querystring');
const parsed = querystring.parse('name=John&age=30');
console.log(parsed);
crypto
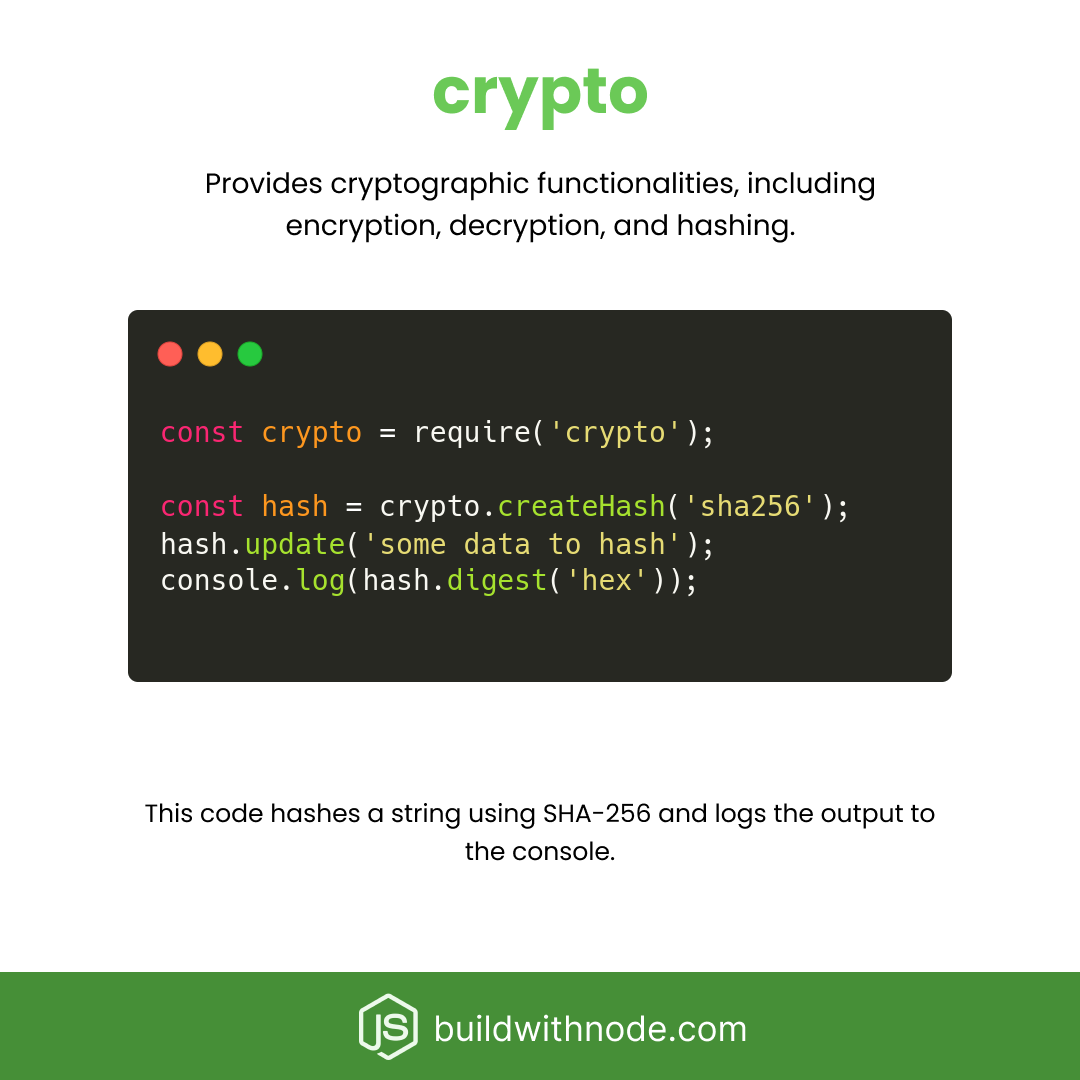
The crypto
module provides lot of cryptographic functionalities that can use for our Nodejs application.
It includes a set of wrappers for OpenSSL's hash, HMAC, cipher, decipher, sign, and verify functions. It enables the implementation of security features such as encryption, decryption, and hashing, which are essential for protecting data and ensuring secure communication in applications.
const crypto = require('crypto');
const hash = crypto.createHash('sha256');
hash.update('some data to hash');
console.log(hash.digest('hex'));
url
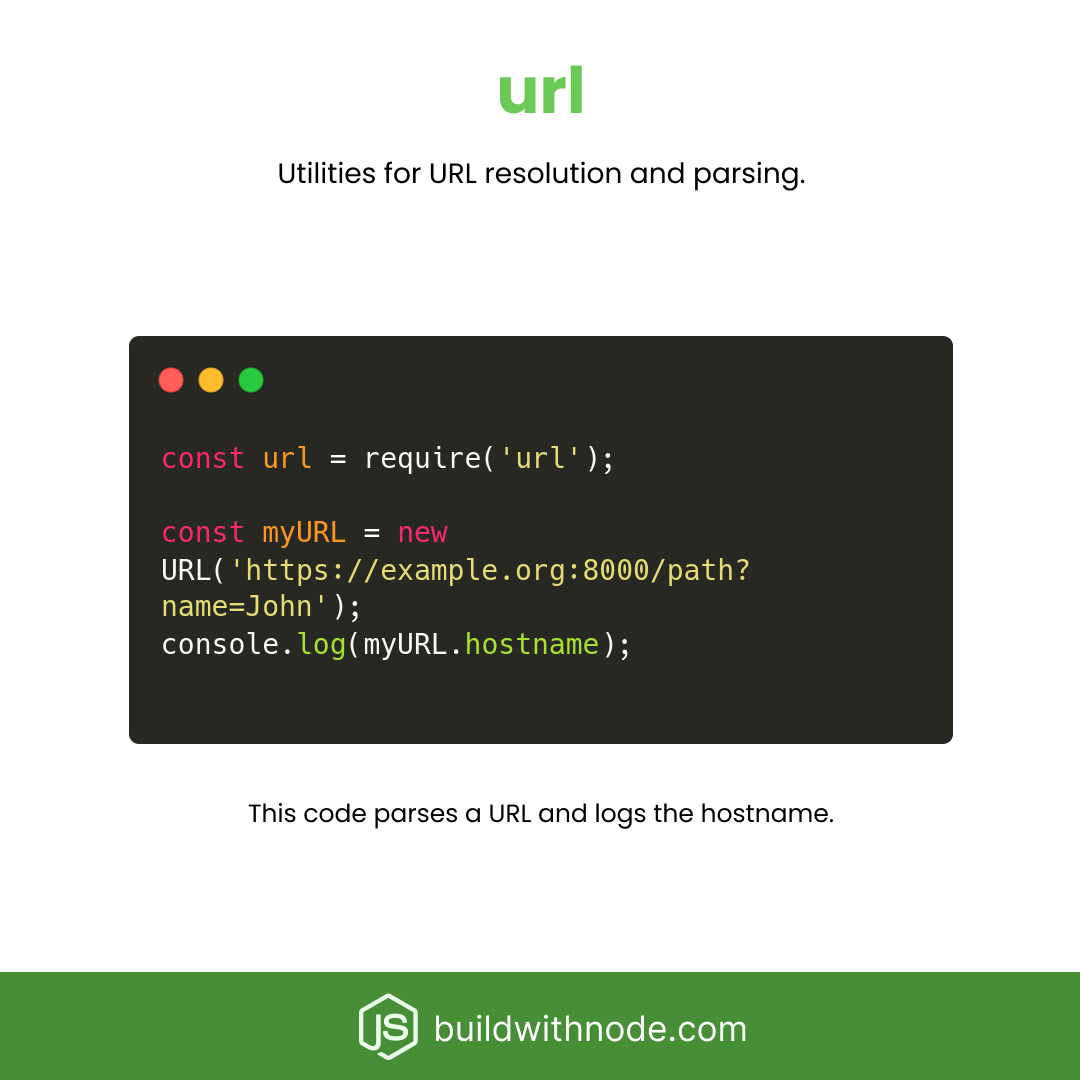
The url
module provides utilities for URL resolution and parsing.
It can parse URLs into their constituent parts (e.g., protocol, hostname, path) and format URL objects into strings. This module is useful for handling and manipulating URLs in web applications, enabling tasks such as URL validation, query parameter extraction, and redirection.
const url = require('url');
const myURL = new URL('https://example.org:8000/path?name=John');
console.log(myURL.hostname);
zlib
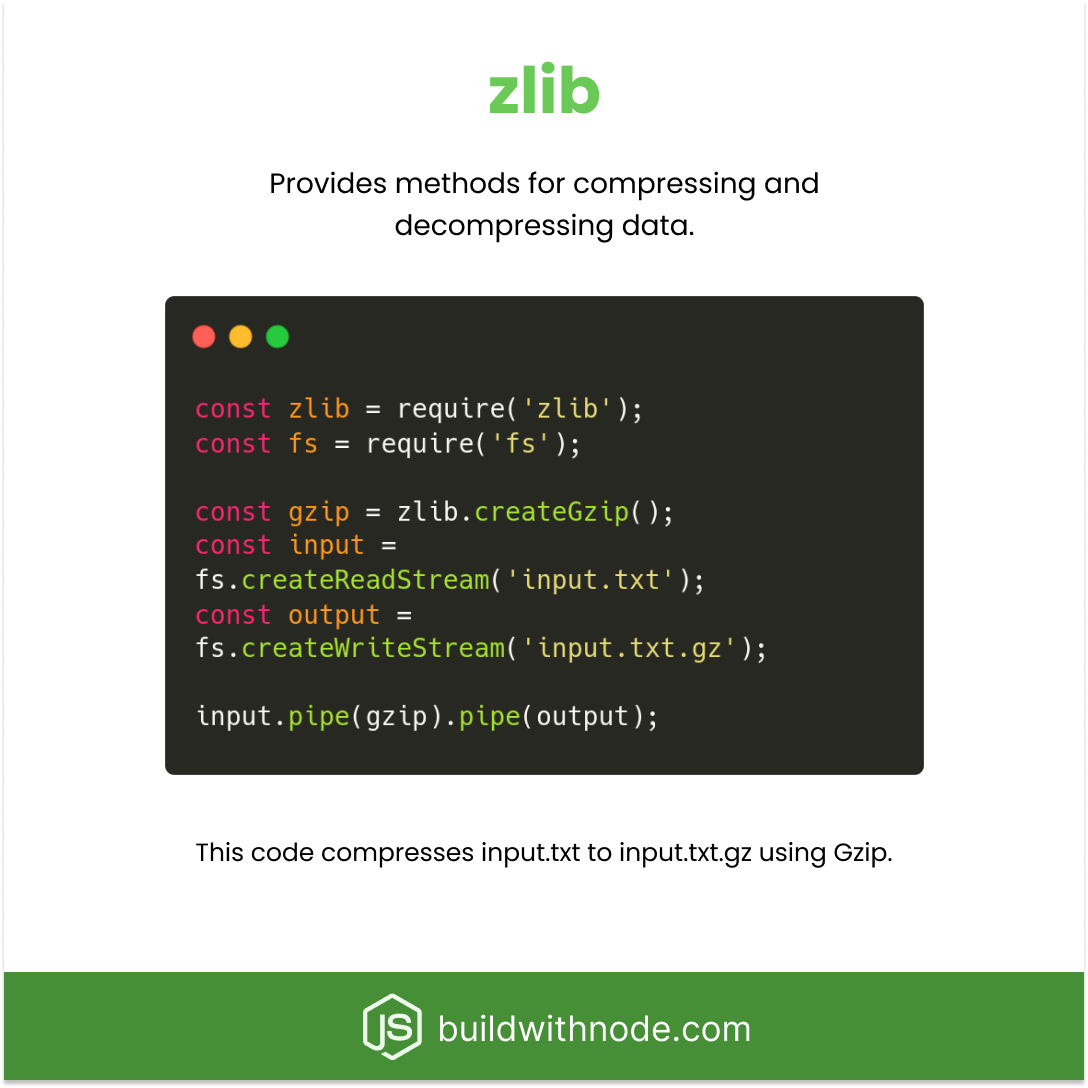
The zlib
module provides methods for compressing and decompressing data.
It use the Deflate, Gzip, and Brotli compression algorithms. It is used to reduce the size of data for storage or transmission, improving performance and efficiency. This module is essential for applications that need to handle large amounts of data, such as file transfer services and web servers.
const zlib = require('zlib');
const fs = require('fs');
const gzip = zlib.createGzip();
const input = fs.createReadStream('input.txt');
const output = fs.createWriteStream('input.txt.gz');
input.pipe(gzip).pipe(output);
dns
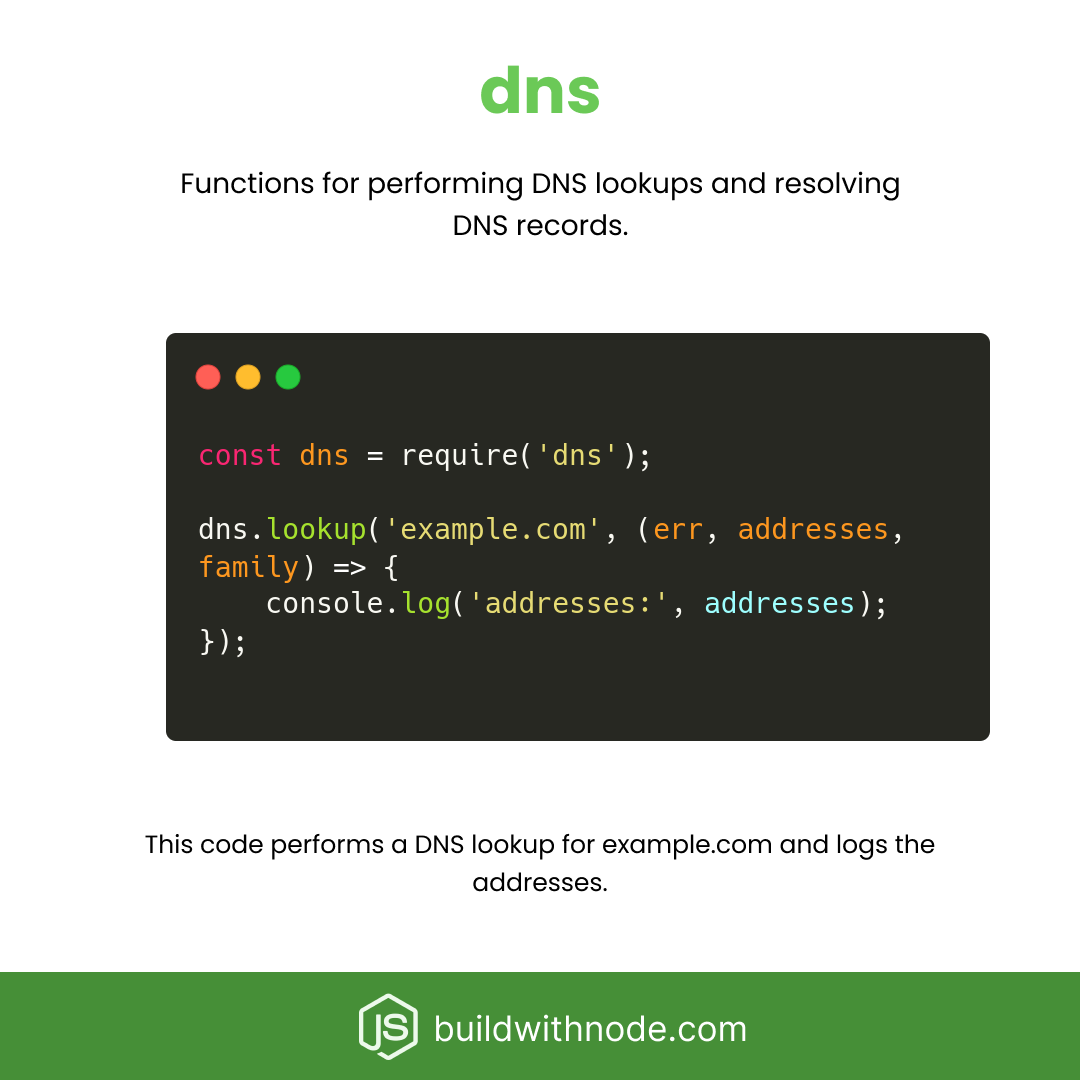
The dns
module provides functions for performing DNS lookups and resolving DNS records.
It can translate domain names into IP addresses and perform various DNS-related queries. This module is useful for network applications that need to resolve domain names, handle DNS-based load balancing, or perform DNS diagnostics.
const dns = require('dns');
dns.lookup('example.com', (err, addresses, family) => {
console.log('addresses:', addresses);
});
child_process
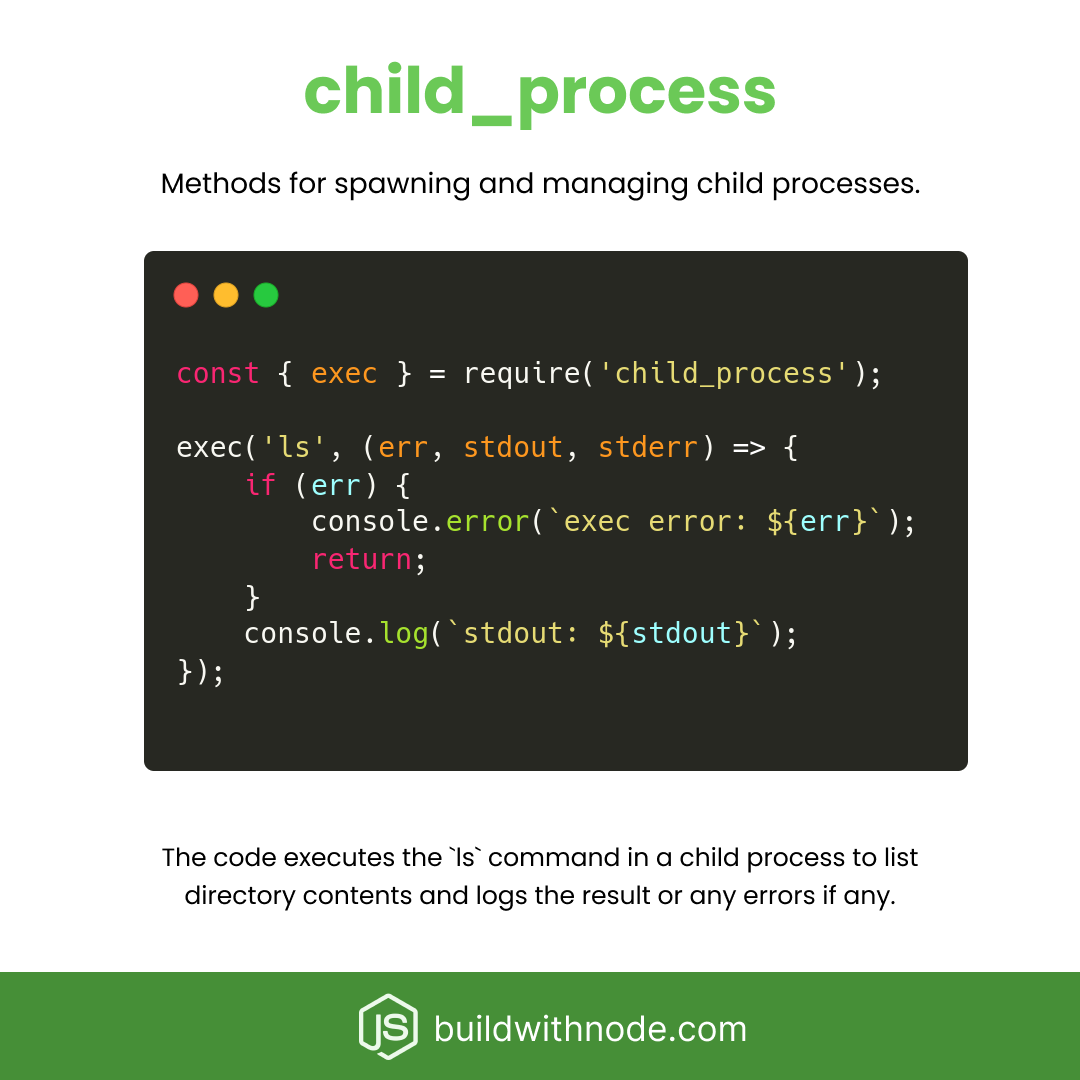
The child_process module allows you to spawn and manage child processes.
It provides methods for executing shell commands, spawning new Node.js processes, and communicating with child processes via standard input, output, and error streams. This module is essential for applications that need to perform parallel processing, execute external commands, or manage multiple processes.
const { exec } = require('child_process');
exec('ls', (err, stdout, stderr) => {
if (err) {
console.error(`exec error: ${err}`);
return;
}
console.log(`stdout: ${stdout}`);
});
cluster
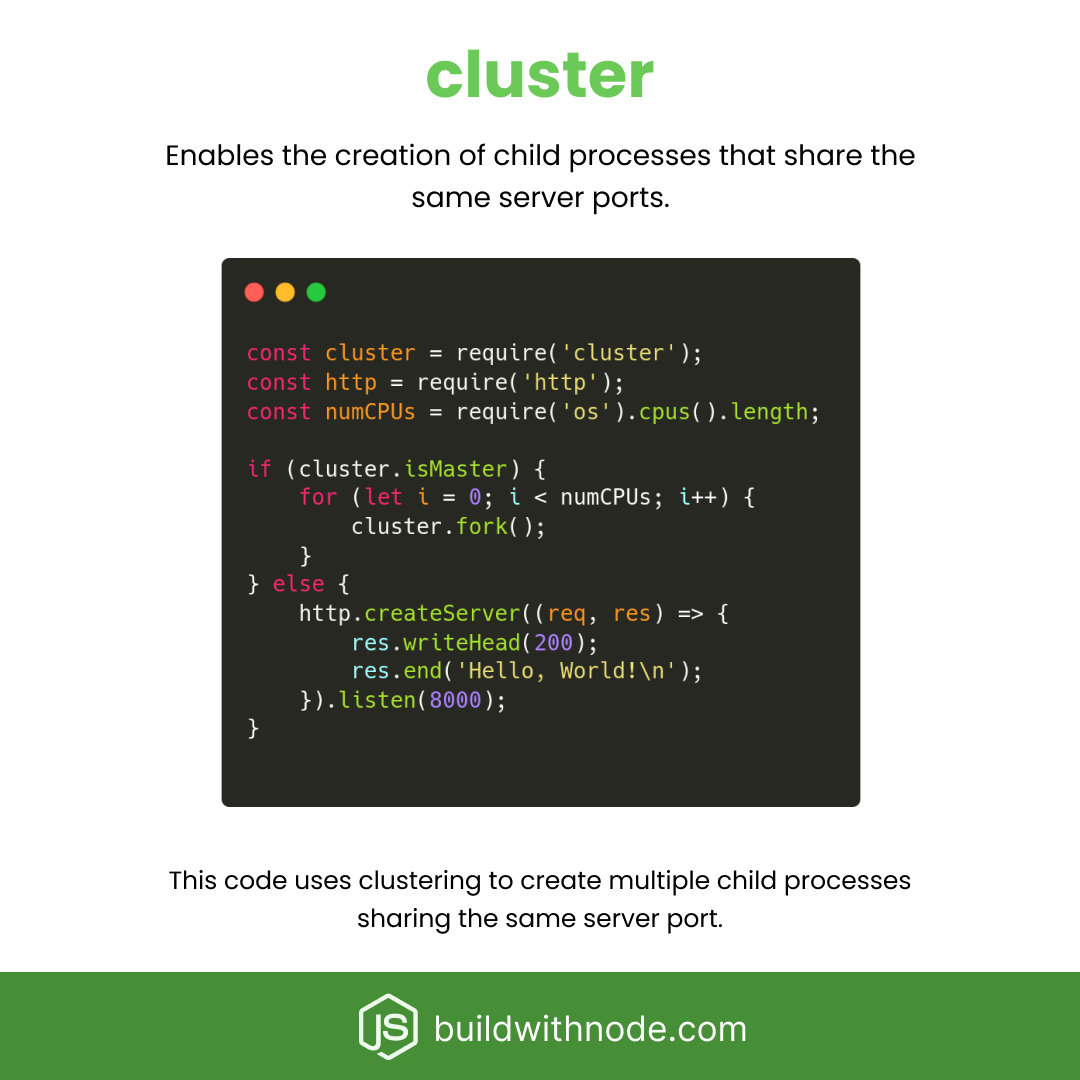
The cluster
module enables the creation of child processes that share the same server ports, allowing for the distribution of workloads across multiple CPU cores.
It simplifies the development of scalable server applications by enabling the creation of worker processes that can handle requests concurrently. This module is particularly useful for improving the performance and reliability of web servers.
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello, World!\n');
}).listen(8000);
}
timers
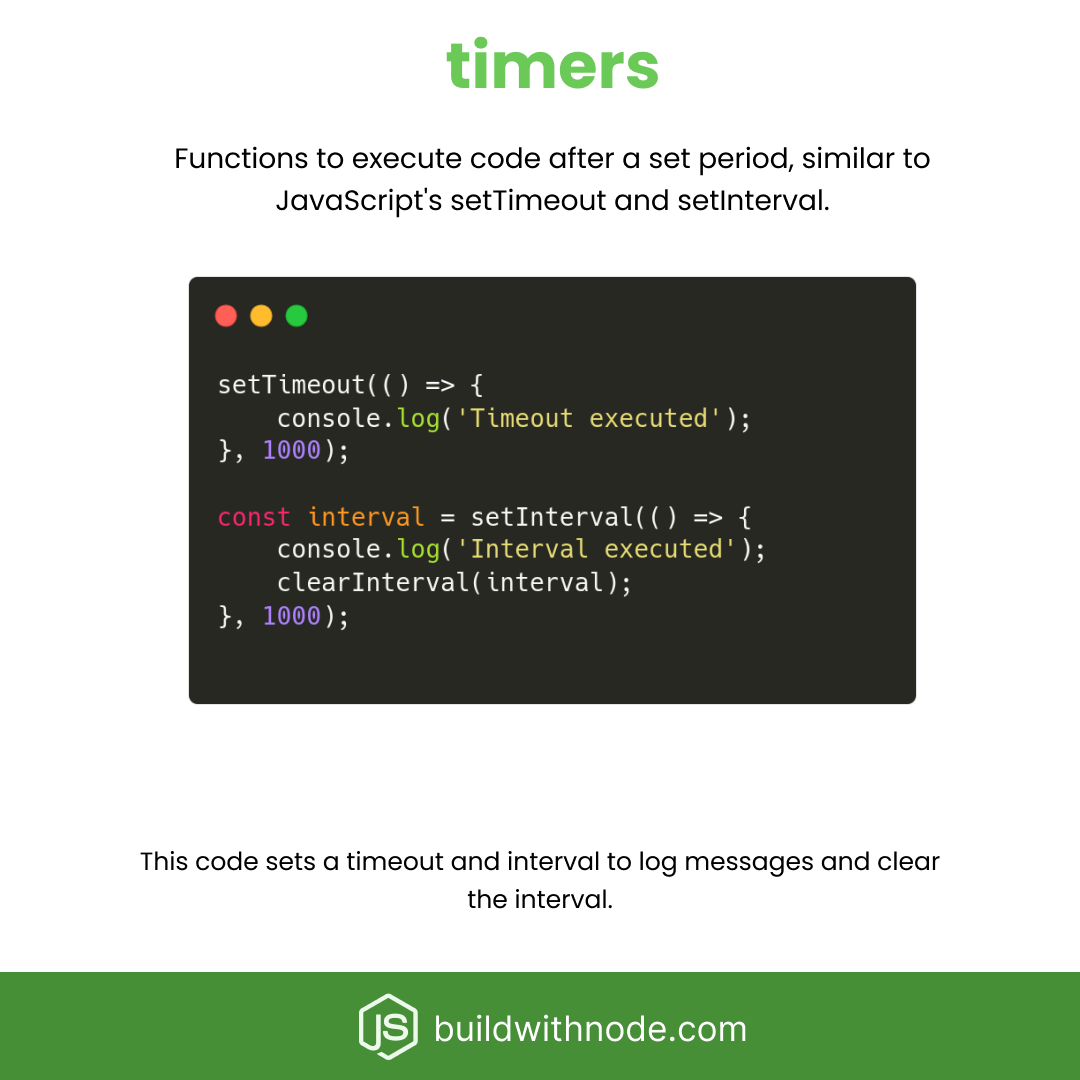
The timers
module provides functions to execute code after a set period, similar to JavaScript's setTimeout
and setInterval
.
It allows scheduling functions to run at specific intervals or after a delay, which is useful for tasks such as polling, periodic updates, and timeout management in asynchronous programming.
setTimeout(() => {
console.log('Timeout executed');
}, 1000);
const interval = setInterval(() => {
console.log('Interval executed');
clearInterval(interval);
}, 1000);
net
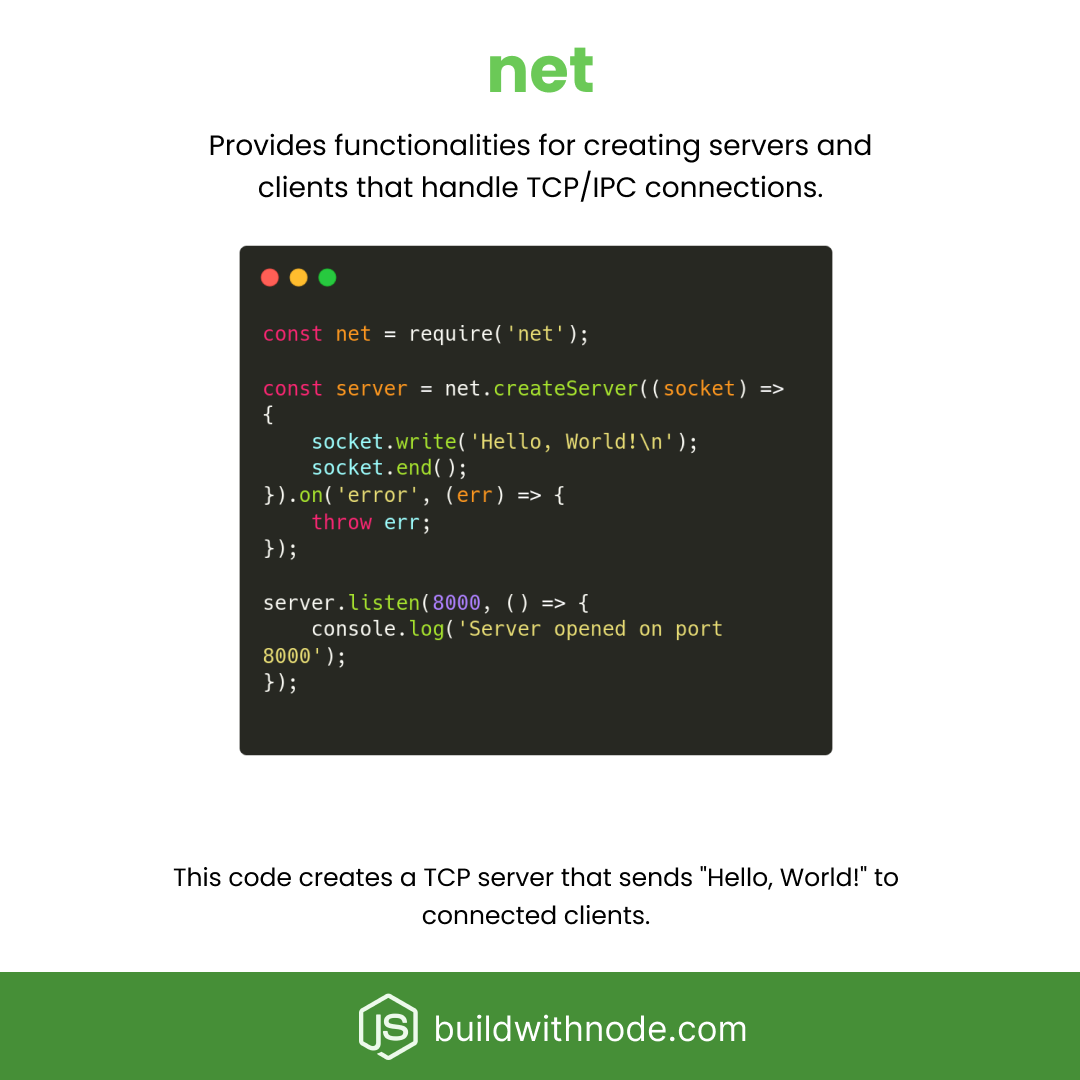
The net
module provides functionalities for creating servers and clients that handle TCP/IPC connections. It enables the development of network applications that require low-level network communication, such as chat servers, file transfer services, and real-time data streaming applications. This module provides a foundation for building custom network protocols and services.
const net = require('net');
const server = net.createServer((socket) => {
socket.write('Hello, World!\n');
socket.end();
}).on('error', (err) => {
throw err;
});
server.listen(8000, () => {
console.log('Server opened on port 8000');
});
vm
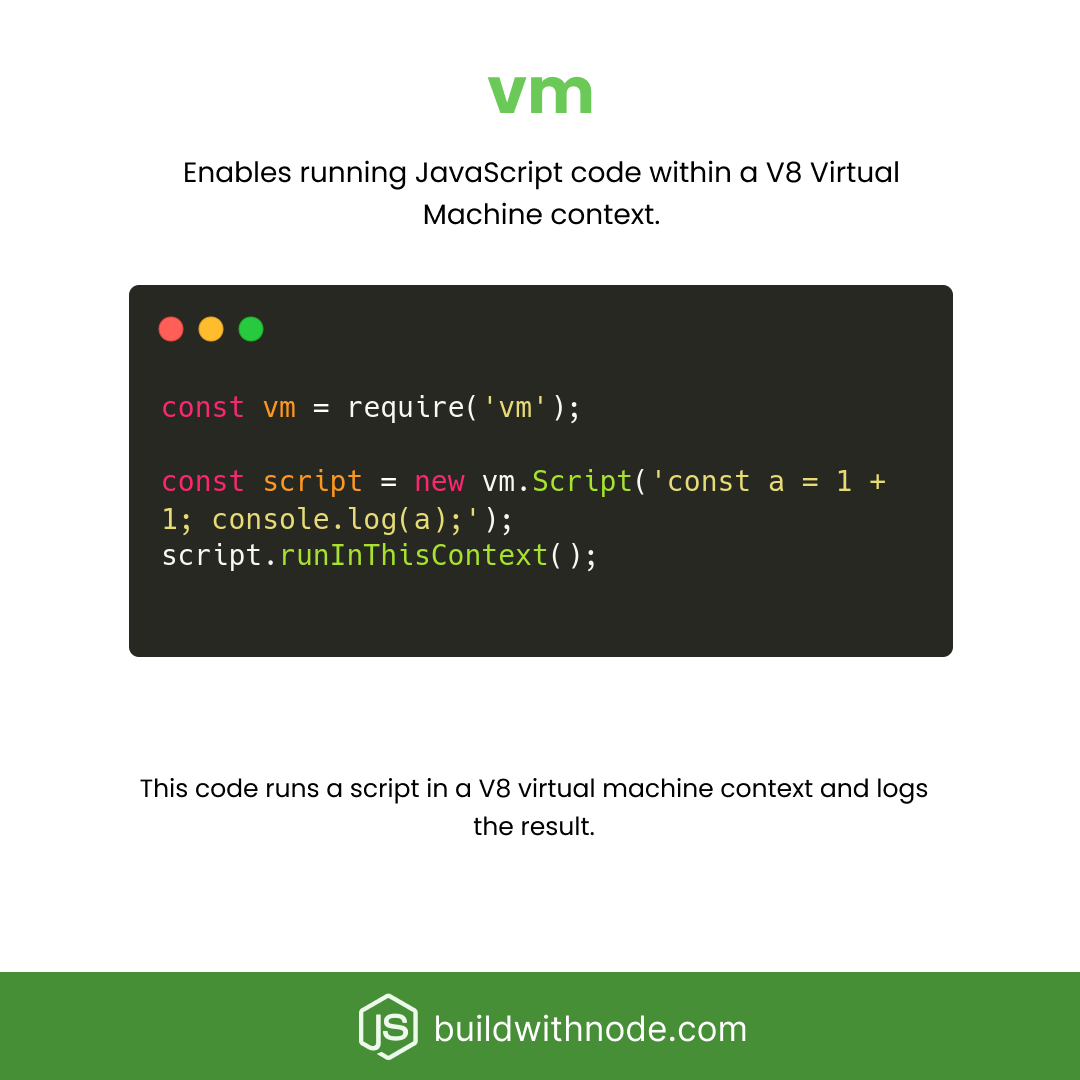
The vm
module enables running JavaScript code within a V8 Virtual Machine context.
It provides methods for creating and managing isolated execution contexts, allowing the execution of untrusted code in a controlled environment. This module is useful for sandboxing, implementing plugin systems, and executing dynamic code safely.
const vm = require('vm');
const script = new vm.Script('const a = 1 + 1; console.log(a);');
script.runInThisContext();
readline
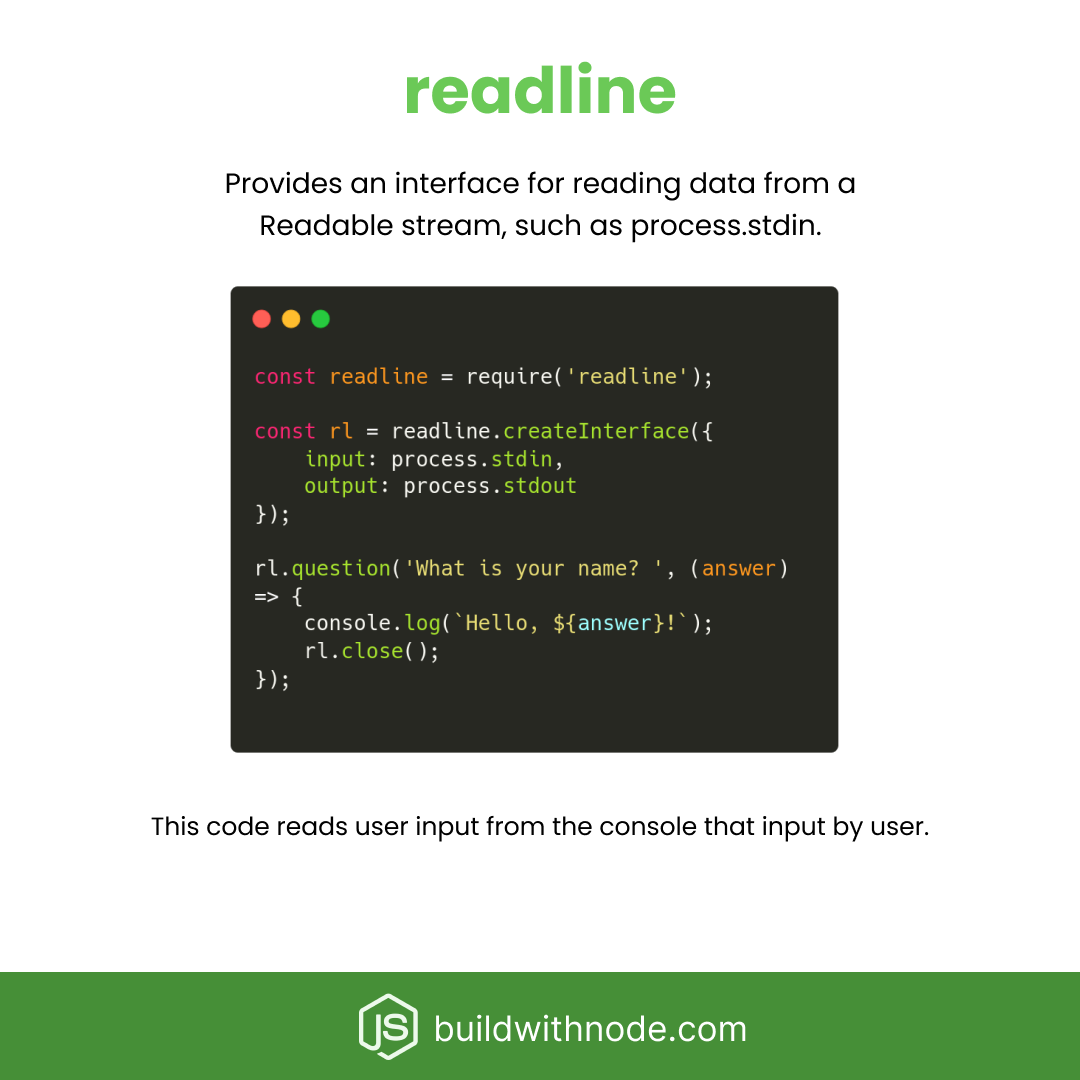
The readline
module provides an interface for reading data from a Readable stream, such as process.stdin. It supports line-by-line reading and provides methods for handling user input interactively, making it suitable for developing command-line interfaces, REPLs (Read-Eval-Print Loops), and other text-based user interactions.